Here is my Arduino Library for the Grove OLED 128x64.
Please help me to optimize it 
github.com/schmron/SSD1308
Features:
- Framebuffer
- Draw simple Geometrics (Pixel, Line, Rectangle, Circle)
- Draw Text
- Change Font
Please use just Arduinos with atmega328 (Framebuffer needs 1kB RAM).
schmron how i can change font size?
For example i need make it double size.
Thanks.
Are there any specific instructions on how to change the font size? I tried changing the #define_width and height but nothing happens. Please reply.
Now we have no idea to change the font of size, but out TFT touch shield v2 can easy do that!
Schmrom’s SSD1308 library at github.com/schmron/SSD1308 seems to have been pulled.
Has anyone hacked a library together to display larger fonts yet? I didn’t find any elsewhere. Looking at the 1308 data sheet, it doesn’t look like there are any built-in fonts, so I guess it would be done by writing parts of double or triple or larger size letters to each page.
I’ll take a stab at this myself, maybe, sooner or later. Need to come up with a bitmap editor …
-w
Does anyone know of a bitmap editor that outputs bitmaps in C/C++ char array? What did Seeed use to create their 1024-byte bitmap for the OLED demo? Sun Micro used to have one long, long ago …
Otherwise, the best I have found is Bitfontmaker (pentacom.jp/pentacom/bitfontmaker2) which allows youto export a font as a row-wise array of 16 bit ints, which can easily be converted into the column-wise 8-bit chars that the OLED chip needs.
OK, you can make your own bitmap with the open source Gimp program and some scripting. Here’s what I did:
-
Draw or import a monochrome image into Gimp. Set the canvas size to 128 cols by 64 rows.
-
Export the image as a PGM (my copy of Gimp doesn’t seem to be capable of PBM.) PGM has the following format: Two lines of metadata, a third line with the number of cols and number of rows (“128 64”) and successive lines that are rowwise byte by byte pixels in the image. The lines will be either 255 (white) or 0 (black).
-
We have to read the rowwise data and convert it into the “pages” the OLED controller wants. There are 8 pages, with 128 bytes in each page. Each byte is a single column “stack” 8 bits high. (See the data sheet.) Here is quick and dirty perl program that will read the PGM file and output an array of chars you can paste into your C++ program:
[code]#!/usr/bin/perl
$junk = ;
$junk = ;
print $junk;
$junk = ();
print $junk;
chomp $junk;
($cols, $rows, $junk) = split(/\s+/, $junk);
$nbytes = $rows * $cols / 8;
for ($r = 0; $r < $rows; $r++) {
for ($c = 0; $c < $cols; $c++) {
$data[$r][$c] = ;
chomp($data[$r][$c]);
if ($data[$r][$c] > 128) { $data[$r][$c] = 1 }
}
}
PGM: 255 is white, 0 is black
print “// $nbytes bytes in $r rows $c cols\n”;
print “static unsigned char bitmap[] PROGMEM = {\n”;
read 8 pages of columns of 8 bits. Each page is 128 bytes.
LSB of each byte is the “top” of the 8 bits of the column
for ($page = 0; $page < 8; $page++) {
for ($c = 0; $c < $cols; $c++) {
$byte[$page][$c] = $data[$page8 + 7][$c] * 128 +
$data[$page8 + 6][$c] * 64 +
$data[$page8 + 5][$c] * 32 +
$data[$page8 + 4][$c] * 16 +
$data[$page8 + 3][$c] * 8 +
$data[$page8 + 2][$c] * 4 +
$data[$page8 + 1][$c] * 2 +
$data[$page8 + 0][$c] ;
print "$byte[$page][$c], "
}
print “\n”;
}
print "\n};\n ";
[/code]
You send the script the PGM file on stdin, formatting it helps a little: “./pgmtoh -d < test2.pgm | fmt”. Paste the array into your program, use the drawBitmap function form the OLED examples.
Hope someone finds this useful. Fonts will be a lot harder. The only font that comes with the library is only 8 pixels high and very hard to read. Still looking for a source for 16 and 32 bit high bitmap fonts. I can do this one character at a time with GIMP, but it will be tedious.
![]()
Well, I got a 32-bit high font loaded, but it’s a kludge, mostly because it is slow. (SeeedOled.sendData sends data one char at a time.)
The chars are 20 bits wide. The PROGMEM pragma is not happy about that, as far as I can tell, I have to load the font data into RAM.
It will be faster to render the font into a full 64x128 bitmap, and then send the bitmap all at once. Working on it.
(Ignore what looks like shadowing in the picture, it is a side effect of an “HD” picture and camera shake.
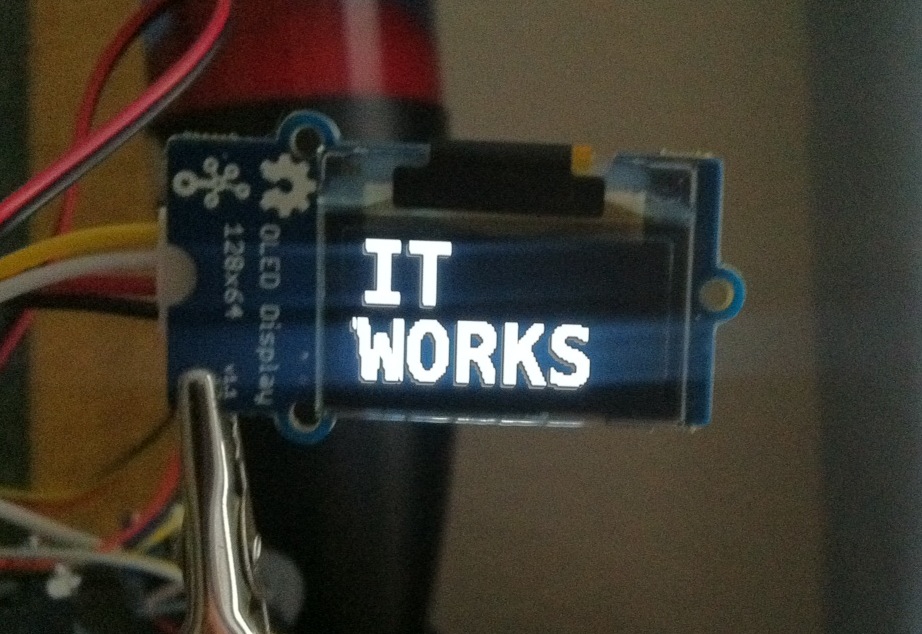
Here’s more info and sources for my 32-bit-high OLED font:
wsanders.net/index.php?entry=entry131009-141306